ChatGPTは、ゲームを作成することができます。
今回は、HTMLとJavaScriptでゲームをつくってもらい、このページ上で遊べるようにしたいと思います。
生成AIは、嘘の情報を生成したり、誤った情報をネット上から拾って回答している場合もあるのでご注意ください。
※ 本サイトでは、ChatGPTからの回答の真偽の検証は行っておりません。ご利用は自己責任でお願いいたします。
※ブラウザによって一部機能が制限されている場合があります。
生成AIを利用する際の注意(再掲載)
・個人情報やプライバシーに関する情報、機密情報を入力しない(情報漏えい対策)
・誤回答、倫理上不適切な回答に注意する(情報の真偽は自己判断)
・著作権の侵害につながるような使用は避ける(特に作品作り)
・生成AIを利用した旨や生成AIから引用をしている旨を明示する
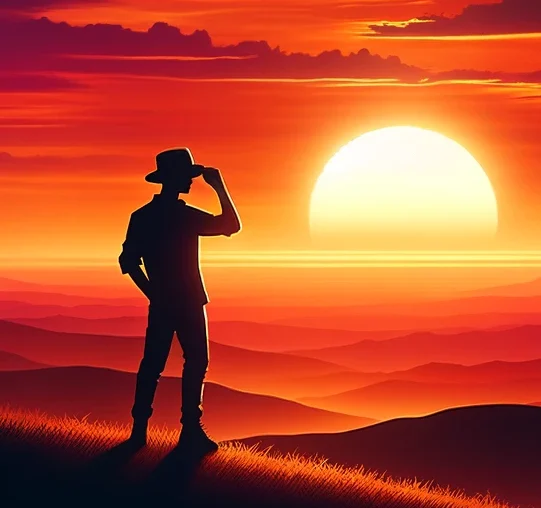
私
あなたは、プログラマーです。
ブラウザ上で遊べるゲームをHTMLファイル内に記述して作成してください。
例1)数当てゲーム
→ ゲームを試す!
以下コード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>数当てゲーム</title>
<style>
input, button {
margin: 10px;
}
</style>
</head>
<body>
<h1>数当てゲーム</h1>
<p>1から100の間の数字を当ててください。</p>
<input type="number" id="guessInput" min="1" max="100">
<button onclick="checkGuess()">予想する</button>
<button onclick="resetGame()">リセット</button>
<p id="message"></p>
<script>
let randomNumber = Math.floor(Math.random() * 100) + 1;
let attempts = 0;
function checkGuess() {
let userGuess = document.getElementById('guessInput').value;
let message = '';
if (userGuess < 1 || userGuess > 100) {
message = '1から100の間の数字を入力してください。';
} else {
attempts++;
if (userGuess < randomNumber) {
message = 'もっと大きい数字です。';
} else if (userGuess > randomNumber) {
message = 'もっと小さい数字です。';
} else {
message = 'おめでとうございます! ' + attempts + ' 回で正解しました!';
}
}
document.getElementById('message').innerText = message;
}
function resetGame() {
randomNumber = Math.floor(Math.random() * 100) + 1;
attempts = 0;
document.getElementById('guessInput').value = '';
document.getElementById('message').innerText = '';
}
</script>
</body>
</html>
例2)じゃんけんゲーム
→ ゲームを試す!
※連打はしないでください
以下コード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>じゃんけんゲーム</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
}
button {
margin: 10px;
padding: 10px 20px;
font-size: 16px;
}
p {
font-size: 18px;
}
</style>
</head>
<body>
<h1>じゃんけんゲーム</h1>
<p id="intro">最初はグー、じゃんけん...</p>
<button onclick="play('グー')">グー</button>
<button onclick="play('チョキ')">チョキ</button>
<button onclick="play('パー')">パー</button>
<p id="result"></p>
<script>
function play(userChoice) {
const choices = ['グー', 'チョキ', 'パー'];
const computerChoice = choices[Math.floor(Math.random() * choices.length)];
let result = '';
if (userChoice === computerChoice) {
result = `引き分けです! コンピュータも${computerChoice}を選びました。`;
} else if (
(userChoice === 'グー' && computerChoice === 'チョキ') ||
(userChoice === 'チョキ' && computerChoice === 'パー') ||
(userChoice === 'パー' && computerChoice === 'グー')
) {
result = `あなたの勝ちです! コンピュータは${computerChoice}を選びました。`;
} else {
result = `あなたの負けです。コンピュータは${computerChoice}を選びました。`;
}
document.getElementById('result').innerText = result;
setTimeout(resetGame, 3000);
}
function resetGame() {
document.getElementById('result').innerText = '';
document.getElementById('intro').innerText = '最初はグー、じゃんけん...';
}
</script>
</body>
</html>
例3)ブラックジャックゲーム
※カードを引いて21に近づけるブラックジャックゲームです。
→ ゲームを試す!
以下コード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ブラックジャックゲーム</title>
<style>
body {
font-family: Arial, sans-serif;
padding: 50px;
}
button {
margin: 10px;
padding: 10px 20px;
font-size: 16px;
}
p {
font-size: 18px;
}
</style>
</head>
<body>
<h1>ブラックジャックゲーム</h1>
<p id="message">カードを引いてください。</p>
<button id="drawButton" onclick="drawCard()">カードを引く</button>
<button onclick="endGame()">終了</button>
<p id="cards"></p>
<p id="score"></p>
<script>
let deck = [];
let playerCards = [];
let playerScore = 0;
function initializeDeck() {
deck = [];
const suits = ['♠', '♥', '♦', '♣'];
const values = ['2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A'];
for (let suit of suits) {
for (let value of values) {
deck.push({suit, value});
}
}
deck = shuffle(deck);
}
function shuffle(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[array[i], array[j]] = [array[j], array[i]];
}
return array;
}
function drawCard() {
if (deck.length === 0) {
initializeDeck();
}
const card = deck.pop();
playerCards.push(card);
updateScore(card);
displayCards();
checkScore();
}
function updateScore(card) {
let cardValue = card.value;
if (cardValue === 'J' || cardValue === 'Q' || cardValue === 'K') {
cardValue = 10;
} else if (cardValue === 'A') {
cardValue = (playerScore + 11 <= 21) ? 11 : 1;
} else {
cardValue = parseInt(cardValue);
}
playerScore += cardValue;
document.getElementById('score').innerText = `得点: ${playerScore}`;
}
function displayCards() {
const cardText = playerCards.map(card => `${card.suit}${card.value}`).join(' ');
document.getElementById('cards').innerText = `カード: ${cardText}`;
}
function checkScore() {
if (playerScore === 21) {
document.getElementById('message').innerText = 'おめでとうございます!21で勝ちました!';
disableDrawButton();
} else if (playerScore > 21) {
document.getElementById('message').innerText = '残念!21を超えました。';
disableDrawButton();
}
}
function endGame() {
document.getElementById('message').innerText = 'ゲームを終了しました。リセットします...';
disableDrawButton();
setTimeout(resetGame, 3000);
}
function disableDrawButton() {
document.getElementById('drawButton').disabled = true;
}
function resetGame() {
playerCards = [];
playerScore = 0;
document.getElementById('cards').innerText = '';
document.getElementById('score').innerText = '得点: 0';
document.getElementById('message').innerText = 'カードを引いてください。';
document.getElementById('drawButton').disabled = false;
initializeDeck();
}
window.onload = () => {
initializeDeck();
};
</script>
</body>
</html>
例4)あっち向いてホイ
→ ゲームを試す!
以下コード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>あっち向いてホイ</title>
<style>
button {
margin: 10px;
padding: 10px 20px;
font-size: 16px;
}
#result {
margin-top: 20px;
font-size: 18px;
}
</style>
</head>
<body>
<h1>あっち向いてホイ</h1>
<p id="instructions">じゃんけんをしてください:</p>
<p id="result"></p>
<div id="game">
<button onclick="playJanken('グー')">グー</button>
<button onclick="playJanken('チョキ')">チョキ</button>
<button onclick="playJanken('パー')">パー</button>
</div>
<p id="result"></p>
<script>
let gameStage = 'janken';
let jankenWinner = null;
function playJanken(playerChoice) {
if (gameStage !== 'janken') return;
const choices = ['グー', 'チョキ', 'パー'];
const computerChoice = choices[Math.floor(Math.random() * choices.length)];
let resultMessage = `あなた: ${playerChoice}, コンピュータ: ${computerChoice} - `;
if (playerChoice === computerChoice) {
resultMessage += '引き分けです。再度じゃんけんしてください。';
document.getElementById('result').innerText = resultMessage;
return;
}
jankenWinner = (playerChoice === 'グー' && computerChoice === 'チョキ') ||
(playerChoice === 'チョキ' && computerChoice === 'パー') ||
(playerChoice === 'パー' && computerChoice === 'グー') ? 'player' : 'computer';
resultMessage += jankenWinner === 'player' ? 'あなたの勝ちです!' : 'あなたの負けです。';
document.getElementById('result').innerText = resultMessage;
gameStage = 'hoi';
setTimeout(() => prepareHoi(), 2000);
}
function prepareHoi() {
document.getElementById('instructions').innerText = 'あっち向いてホイ: 方向を選んでください。';
document.getElementById('game').innerHTML = `
<button onclick="playHoi('上')">上</button>
<button onclick="playHoi('下')">下</button>
<button onclick="playHoi('左')">左</button>
<button onclick="playHoi('右')">右</button>
`;
}
function playHoi(playerDirection) {
if (gameStage !== 'hoi') return;
const directions = ['上', '下', '左', '右'];
const computerDirection = directions[Math.floor(Math.random() * directions.length)];
let resultMessage = `あなた: ${playerDirection}, コンピュータ: ${computerDirection} - `;
resultMessage += (playerDirection === computerDirection) ?
(jankenWinner === 'player' ? 'あなたの勝ちです!' : 'コンピュータの勝ちです。') :
'引き分けです。';
document.getElementById('result').innerText = resultMessage;
resetGame();
}
function resetGame() {
gameStage = 'janken';
jankenWinner = null;
setTimeout(() => {
document.getElementById('instructions').innerText = 'じゃんけんをしてください:';
document.getElementById('game').innerHTML = `
<button onclick="playJanken('グー')">グー</button>
<button onclick="playJanken('チョキ')">チョキ</button>
<button onclick="playJanken('パー')">パー</button>
`;
document.getElementById('result').innerText = '';
}, 3000);
}
</script>
</body>
</html>